ABOUT US
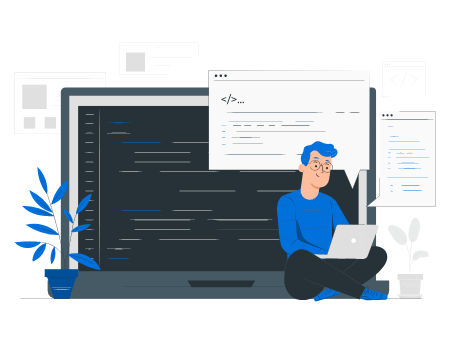
Visual Sort offers an engaging platform to learn sorting algorithms through interactive visualizations. It covers popular algorithms like Bubble Sort, Merge Sort, and more, making complex concepts easy to understand. Ideal for students and educators, it combines education and user-friendly design to enhance learning experiences.